How to Calculate Absolute Value
How to use the built-in function abs:Absolute value (integer type) = abs(<integer type>)
Absolute value (floating-point type) = abs(<floating-point type>)
Absolute value (floating-point type) = abs(<complex type>)
How to use the fabs function from the math module:
import math
Absolute value (floating-point type) = math.fabs(<integer type>)
Absolute value (floating-point type) = math.fabs(<floating-point type>)
Difference between abs function and math.fabs function
- If you pass an integer type to the abs function, it returns the absolute value as an integer type. However, the math.fabs function always returns the absolute value as a floating-point type.
- If you pass a complex type (complex) to the math.fabs function, an error occurs (TypeError: must be a real number, not complex).
math module and cmath module
The math module provides mathematical functions but does not support complex numbers. If you want to handle complex numbers, use the cmath module. However, the cmath module does not provide a function to return the absolute value. To get the absolute value of a complex number, use the abs function.Sample Code
The following sample code calculates and displays the absolute value using the abs function.int_p_value = 99
int_m_value = -99
float_p_value = 99.99
float_m_value = -99.99
print(abs(int_p_value)) # Result = 99
print(abs(int_m_value)) # Result = 99
print(abs(float_p_value)) # Result = 99.99
print(abs(float_m_value)) # Result = 99.99
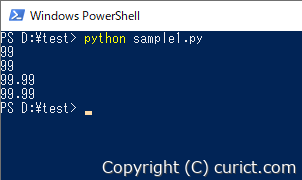
The following sample code calculates and displays the absolute value using the fabs function from the math module.
import math
int_p_value = 99
int_m_value = -99
float_p_value = 99.99
float_m_value = -99.99
print(math.fabs(int_p_value)) # Result = 99.0
print(math.fabs(int_m_value)) # Result = 99.0
print(math.fabs(float_p_value)) # Result = 99.99
print(math.fabs(float_m_value)) # Result = 99.99
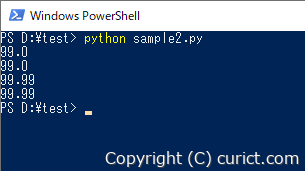
References
- Python Official Documentation - abs - Built-in Functions
- Python Official Documentation - math.fabs - math - Mathematical functions
- Python Official Documentation - cmath - Mathematical functions for complex numbers
Test Environment
- Python 3.11.3 (tags/v3.11.3:f3909b8, Apr 4 2023, 23:49:59) [MSC v.1934 64 bit (AMD64)] on win32
- Microsoft Windows 10 Enterprise Version 22H2 OS Build 19045.3570 Experience: Windows Feature Experience Pack 1000.19052.1000.0