Getting a sequence number with enumerate
sampleList = ['data1', 'data2', 'data3']
for index, value in enumerate(sampleList):
print(f'index = {index} value = {value}')
- In line 1, we create a list.
- In line 3, the sequence number starting from 0 is assigned to ‘index’ and the value of the list is assigned to ‘value’.
- The names ‘index’ and ‘value’ in line 3 can be changed according to your needs.
index = 0 value = data1
index = 1 value = data2
index = 2 value = data3
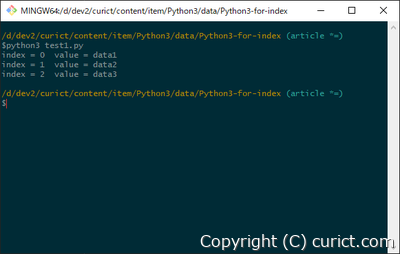
Specifying the initial value of the sequence number
sampleList = ['data1', 'data2', 'data3']
# Start the sequence number from 1
for index, value in enumerate(sampleList, 1):
print(f'index = {index} value = {value}')
- In line 4, you can specify the initial value of the sequence number with the second argument of enumerate.
index = 1 value = data1
index = 2 value = data2
index = 3 value = data3
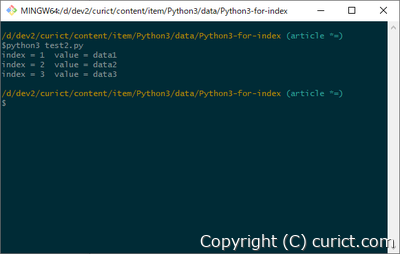
Reference
Test Environment
- Python 3.9.0 (tags/v3.9.0:9cf6752, Oct 5 2020, 15:34:40) [MSC v.1927 64 bit (AMD64)] on win32
- Microsoft Windows 10 Pro Version 20H2 OS Build 19042.844 Experience: Windows Feature Experience Pack 120.2212.551.0